Building Ionic React App
6 years ago -
- Make sure you have Node.js installed (https://nodejs.org/dist/v12.13.0/node-v12.13.0-x86.msi)
- Open command prompt and type in this command to create an app: npx ionic start myApp blank —type=react
- Make sure you have VSCode installed (https://code.visualstudio.com/download)
- cd into myApp
- Type in this command to open the project in VSCode: code .
- Inside App.tsx you can see something like this:
<Route path="/home" component={Home} exact={true} />
This means that when we navigate to /home it shows a page called Home which is located inside pages directory. - Run the project using this command: npx ionic serve
- Switch to the editor and create a new page called List.tsx with this content:
import {
IonContent,
IonHeader,
IonPage,
IonTitle,
IonToolbar,
} from "@ionic/react";
import React from "react";
const List: React.FC = () => {
return (
<IonPage>
<IonHeader>
<IonToolbar>
<IonTitle>This is a sample page</IonTitle>
</IonToolbar>
</IonHeader>
<IonContent className="ion-padding">
{["Item one", "Item two", "Item 3"].map((item) => (
<p key={item}>{item}</p>
))}
</IonContent>
</IonPage>
);
};
export default List;
- Add a new route for the newly created page inside App.tsx right after the
<Route path="/home" component={Home} exact={true} />
:
<Route path="/list" component={List} exact={true} />
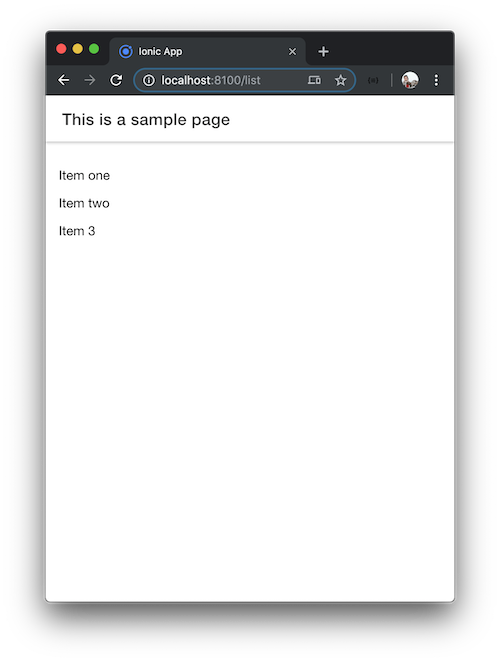